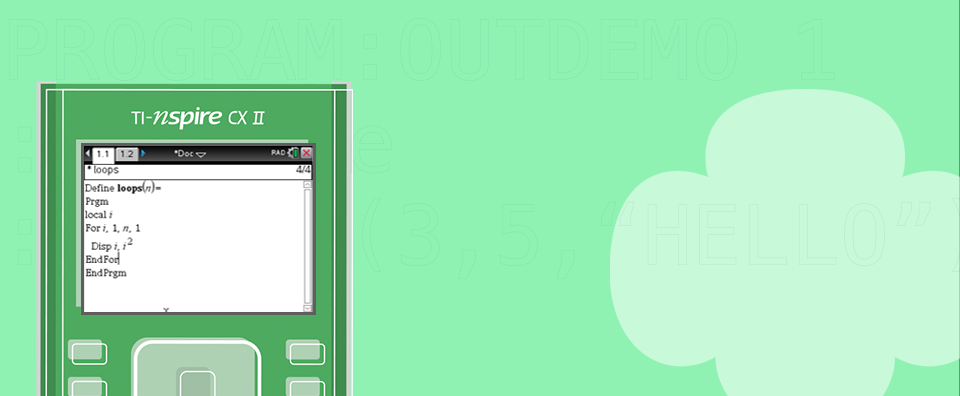
Coding for Good:
TI-Nspire™ CX technology
Choose your level — and let’s start coding to earn
the Coding for Good, Badge 1: Coding Basics
Junior: Coding for Good, Badge 1 >>
Introduction
The programming language BASIC (stands for Beginner’s All-purpose Symbolic Instruction Code) was developed in the 1960s as an easy system for teaching computer programming. TI-Basic is similar to other flavors of BASIC, but you must select the programming words and commands from the onboard menus, as you will soon see.
The TI-Nspire™ CX or TI-Nspire™ CX II graphing calculators can be used at this level.
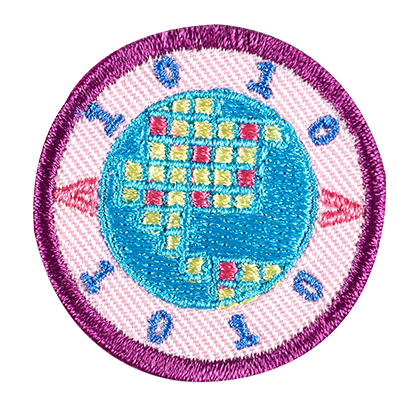
Requirements
Junior Coding for Good, Badge 1 requirements:
1. Create algorithms for a computer that follow a sequence.
2. Use loops to improve your algorithm.
3. Keep your code interesting with conditionals.
4. Create your own set of commands that use conditionals.
5. Learn about women in computer science.
Be sure to review the “Junior Coding for Good” guide for the badge requirements and badge steps provided by the Girl Scouts and your leader. It also includes relevant vocabulary and interesting background information to spark your interest in coding. This lesson will allow you to earn Badge 1: Coding Basics using your TI-Nspire™ CX family graphing calculator.
Step 1
For an introduction to programming on a calculator TI-Nspire™ CX family graphing calculator, see the TI Codes lessons. Units 1 through 4 should be enough to get started, including coverage of algorithms and conditionals as the Badge requirements state. After completing those lessons, you will then have a basic understanding and can return to this lesson for the Junior Coding, Badge 1 project. Note: You will follow the step-by-step instructions given on the webpages, but will actually do the coding on your calculator.
How to navigate: You will navigate through each Skill Builder by clicking on the “Step 1” in the right bottom corner. After you complete all the steps in Skill Builder 1, you will then move to Skill Builder 2, and so on until you complete all of Unit 1. You will then move on to Unit 2, 3, 4 ... and do the same thing.
Step 2
After you have completed the TI Codes Units 1–4, you know about:
- The programming process (planning, coding, testing and debugging)
- Using the TI-Nspire™ Program Editor and its menus
- Running a program
- Some important pieces of TI-Basic code: Input, Disp, variables and assignment statements, If…Then…Else structures and loops, like For and While (some of these statements are shown to the right)
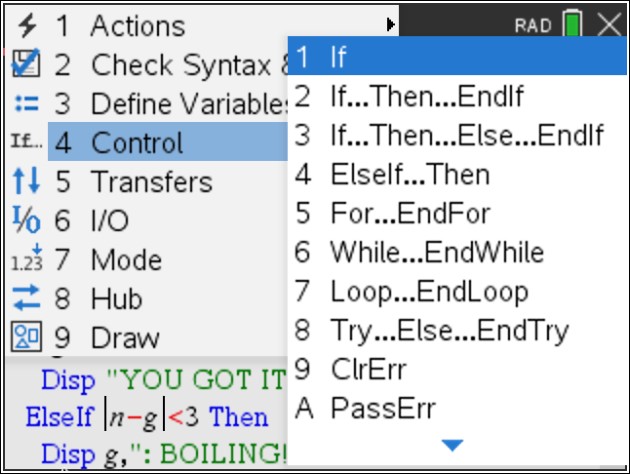
Step 3
Your Badge 1 project will be a Number Guessing Game. The calculator will select a random number between one and 100, and the player must guess the number. And:
- The game continues until the player guesses the number
- If the guess is wrong the computer will tell you whether to guess HIGHER or LOWER to get closer to the number
- After the player guesses the number, the computer will report the number of guesses that the player took
A sample run of the program is shown to the right. The player entered the numbers 45, 34 and 12. The program responded with HIGHER and LOWER. Think about the plan. You will begin writing the program next.
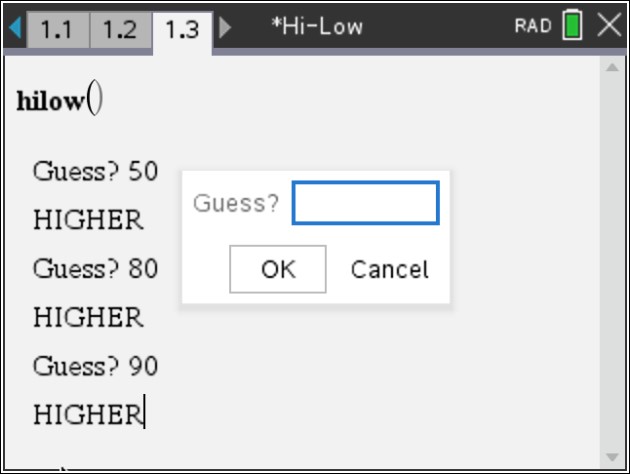
Step 4
Our plan is:
- Create some variables
- Use a While loop to keep guessing as long as we have not guessed the number
- In the loop
- Count the guesses
- Input a guess
- Check to see whether the guess is too high or too low
- After the loop
- Display a congratulations message
- Display the number of guesses
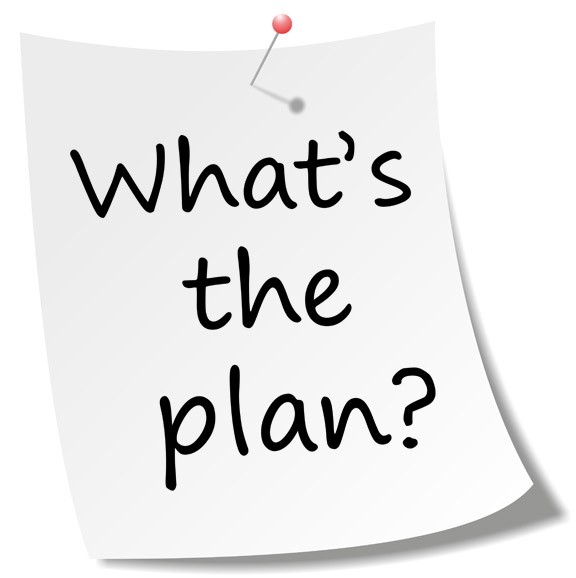
Step 5
Start a new program.
From the Home screen, select New Document Select Program Editor from the menu, name the program HILOW and press [enter]. You are now using the Program Editor. As you learned in the TI Codes lessons, this Editor has its own programming menu.
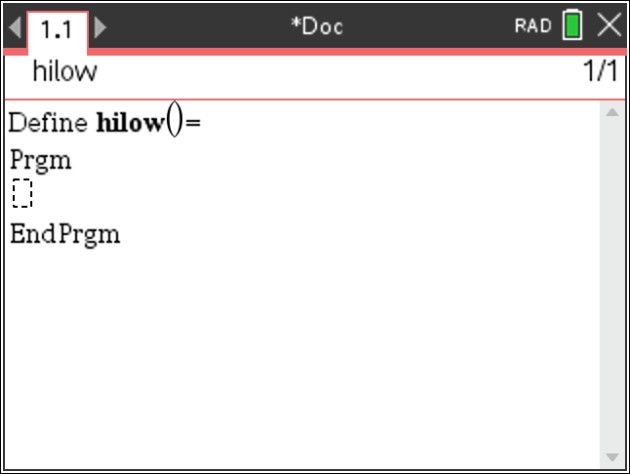
Step 6
Start your program by setting up three variables: The computer’s random number (n), the player’s guess (g) and the guess-counter ©.
Type the variable n and the := symbol found at ctrl-[math templates] to the right of the 9 key.
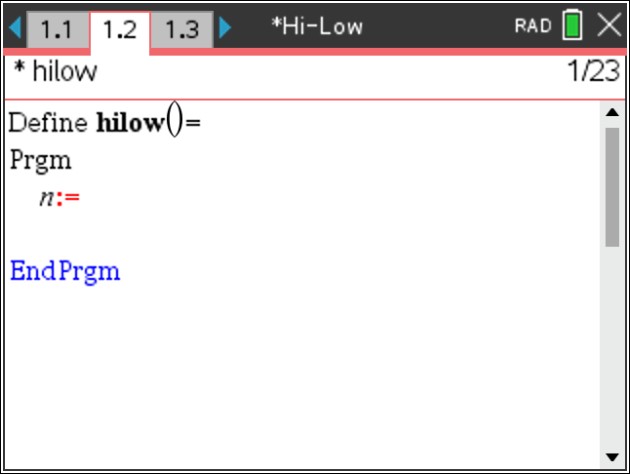
Step 7
Now enter the randInt() function that produces a random integer.
You can simply type randInt() or find it in the [catalog] (the key with the book on it), tab 1. Press the letter R and then scroll (down-arrow) to the randInt() function and press [enter].
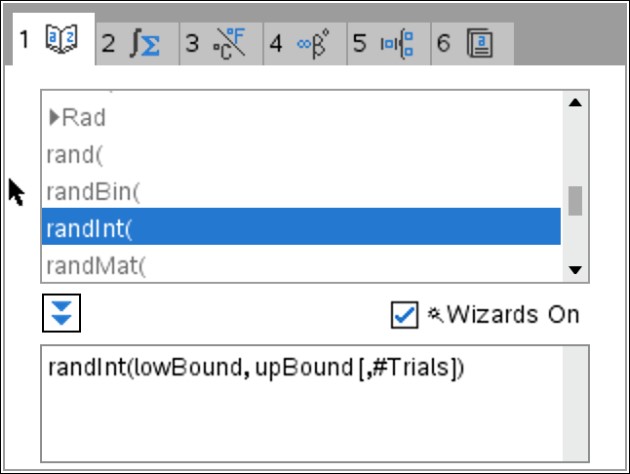
Step 8
Complete the rest of the statement by adding the lower and upper values inside the parentheses:
n := randInt(1, 100)
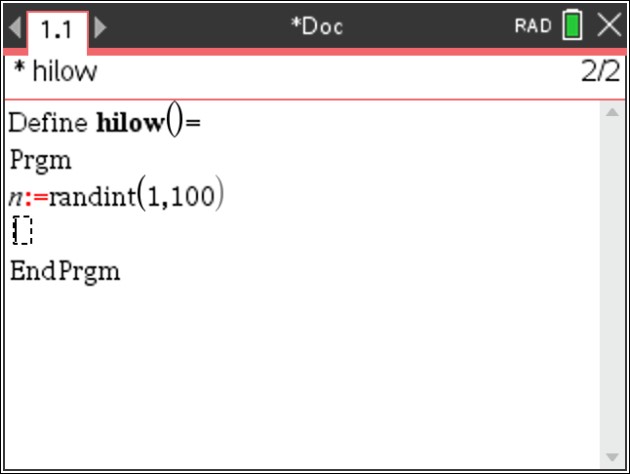
Step 9
The other two variables, g and c are given the value 0. Remember to use := (assignment) and the correct letters.
Your program may contain blank lines and extra spaces (as pictured). This has no effect on the program.
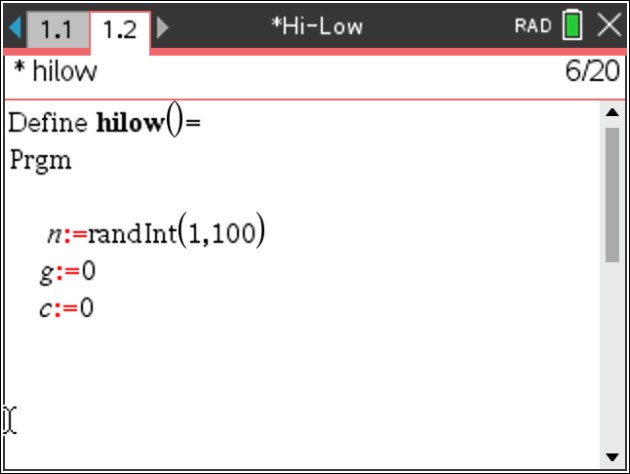
Step 10
Now make a While…EndWhile loop to continue entering guesses until the guess equals the computer’s number (as long as g does not equal n).
See the next step for more information on the While statement.
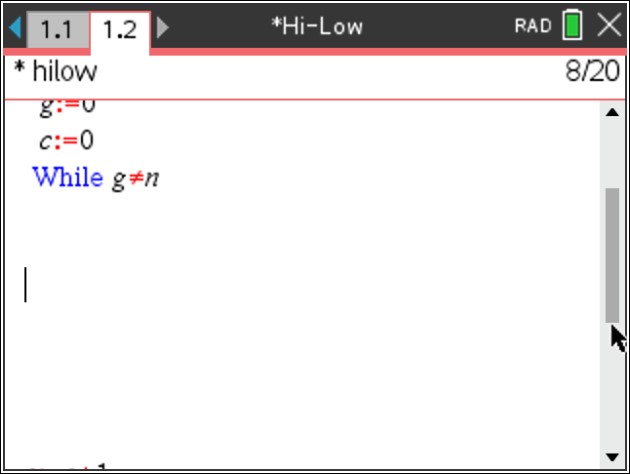
Step 11
While…Endwhile is found on [menu] > Control, and you will type the condition after While.
The “does not equal” symbol is on the [ctrl] [=] menu with the all the other relational operators.
While g ≠ n
EndWhile
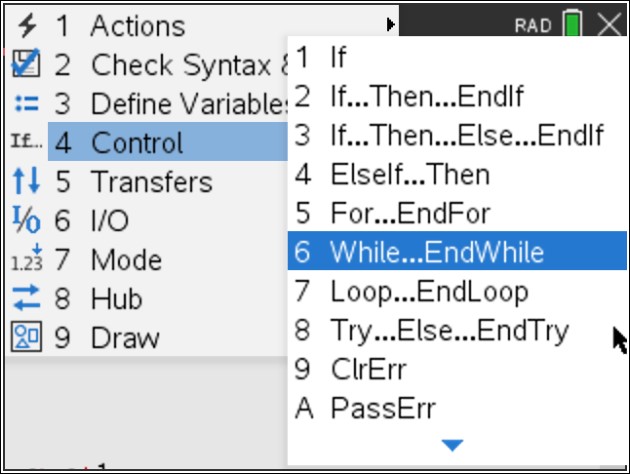
Step 12
Inside the While loop (we have indented the block for clarity):
- Add 1 to the guess-counter variable c.
- Write a Request statement to accept the player’s guess (g).
Request is found on [menu] I/O > Request. After the word Request type
the word “Guess?” in quotes, then a comma,
then the letter g. “Guess?” will appear in
green automatically.
- “Guess?” is the prompt , g is the variable.
- Now add two If statements:
- If g is less than (<) the number (n) – display “HIGHER”
- If g is greater than (>) the number (n) – display “LOWER”
- You can simply type the word If or get it from [menu] > Control.
- Be sure to write two If… statements even though only one is shown here.
Reminder: The [ctrl] [=] menu has the symbols <, >.
Note 1: The indentations in the code are for clarity only. Indentations have no effect on the program and are optional, but useful for debugging.
Note 2: if you want UPPERCASE letters use the [shift] key, or use [ctrl] [shift] for caps lock.
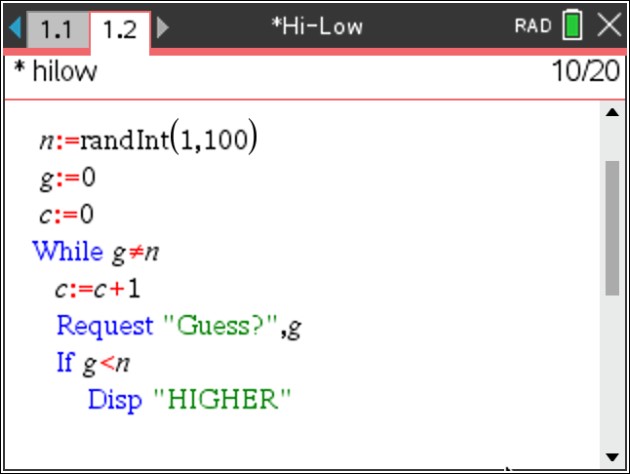
Step 13
After the EndWhile statement:
- Display “YOU GOT IT!” and
- Display the number of guesses (c) that the player took to guess the number
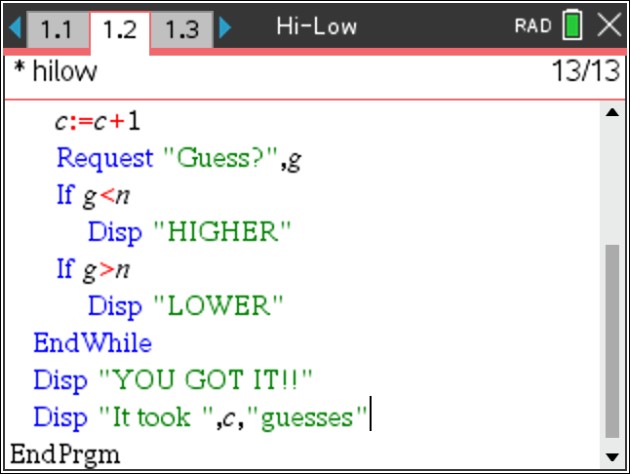
Step 14
To run the program, press ctrl+R to paste the name of the program on a Calculator page, and press [enter] to begin running the program.
When the program ends (see Done) you can return to the Program Editor page by pressing [ctrl]-[leftarrow] (previous page), and make changes as needed.
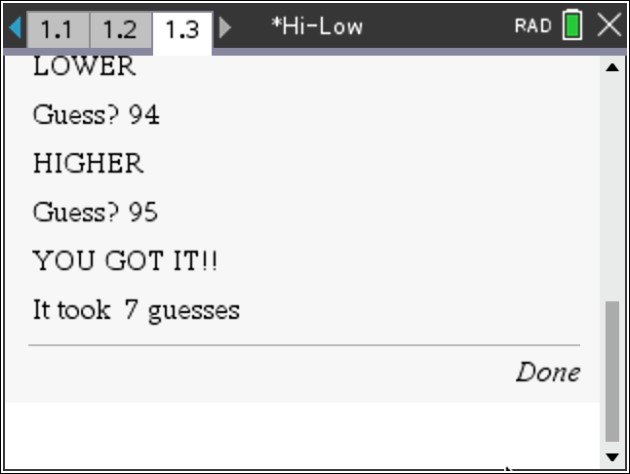
Step 15
For the final part of the badge requirement, remember to research “women in computer science,” such as Margaret Hamilton, who helped humans land on the moon. Use the internet for your searching.
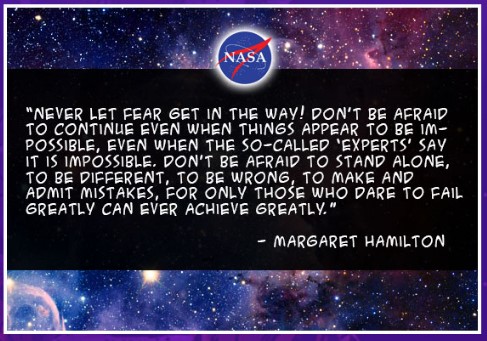
Step 16
Congratulations!
You have completed the requirements for earning your Junior Coding for Good, Badge 1: Coding Basics. Now that you have completed this requirement, you are challenged to give service by sharing what you have learned about coding with others. Refer to the “Coding for Good” Girl Scout guide for suggestions on how to do so.
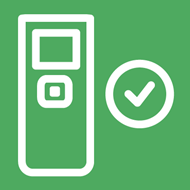
Step 17
What’s next? (Optional extensions)
Ready to try some additional practices with your new skills? 1. Try these to edit your game from above:
- Before the game begins, let the player enter the upper number (100 in the lessons) to play with. Input T and use randInt(1,T) instead of randInt(1,100).
- “Trickster”: Want to play a trick on the player? After each guess, have the program pick a new random number. Do you think the player will ever get the number?
- “Warm or Cold”: Guess the number from one to 100 again, but this time, the computer responds with “Freezing-Cold-Warm-Hot-Boiling” depending on how close the guess is to the answer. You can decide just what each of those words means in your code, but do not tell the player.
Step 18
Optional extensions (continued):
2. Whitney Wolfe Herd is an American entrepreneur. She is founder and CEO of Bumble, a popular social and dating app. Bumble launched in 2014 and now the company is now valued at more than 1 billion dollars.
- How much is 1 billion (1,000,000,000)? How long is 1 billion seconds in days and years? Use the calculator. How long will it take to spend 1 billion dollars? Write a program to let the user enter the amount she spends in one day and display 1) how many days and 2) how many years it will take to spend 1 billion dollars.
3. Did you know you can create other “graphical” games on your calculator? Take a peek at the projects “SNAKE” or the “MAZE” in the Beyond Basics projects to try your hand at a few simple games.
Warning: These are coded using TI-Basic for the TI-84 Plus family of graphing calculators, but the languages are very similar. The projects are more complex, but would be an excellent challenge!
Cadette: Coding for Good, Badge 1 >>
Introduction
The programming language BASIC (stands for Beginner’s All-purpose Symbolic Instruction Code) was developed in the 1960s as an easy system for teaching computer programming. TI-Basic is similar to other flavors of BASIC, but you must select the programming words and commands from the onboard menus, as you will soon see.
The TI-Nspire™ CX II graphing calculator is required at this level.
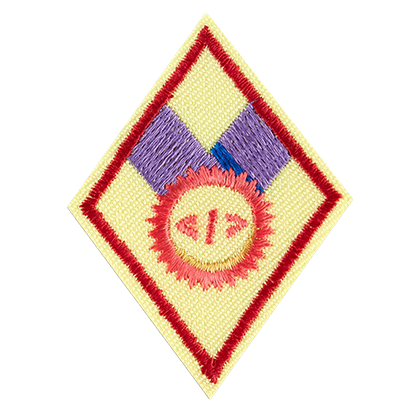
Requirements
Cadette Coding for Good, Badge 1:
meme, functions, pseudocode, shareable
1. Learn about functions and arguments.
2. Explore how memes are created.
3. Write pseudocode for a meme.
4. Write shareable code.
5. Share your meme.
Be sure to review the “Cadette Coding for Good” guide for the badge requirements and badge steps provided by your leader and the Girl Scouts. It also includes relevant vocabulary and interesting background information to spark your interest in coding. This lesson will allow you to earn the Badge 1: Coding Basics using your TI-Nspire™ CX II graphing calculator.
Step 1
For an introduction to programming on a calculator TI-Nspire™ CX family graphing calculator, see the TI Codes lessons. Units 1 through 4, & Unit 6 (Drawing) should be enough to get started, including coverage of algorithms and conditionals as the Badge requirements state. After completing those lessons, you will then have a basic understanding and can return to this lesson for the Cadette Coding, Badge 1 project. Note: You will follow the step-by-step instructions given on the webpages, but will actually do the coding on your calculator.
How to navigate: You will navigate through each Skill Builder by clicking on the “Step 1” in the right bottom corner. After you complete all the steps in Skill Builder 1, you will then move to Skill Builder 2, and so on until you complete all of Unit 1. You will then move on to Unit 2, 3, 4 ... and do the same thing.
Step 2
After you have completed the TI Codes Units 1–4, & Unit 6, you know about:
- The programming process (planning, coding, testing and debugging)
- Using the TI-84 Program Editor and its menus
- Running a program
- Some important pieces of TI-Basic code: Input, Disp, variables and assignment statements, If…Then…Else structures and loops, like For and While (some of these statements are shown to the right)
- Draw features in the programming world of TI-Nspire™ CX II
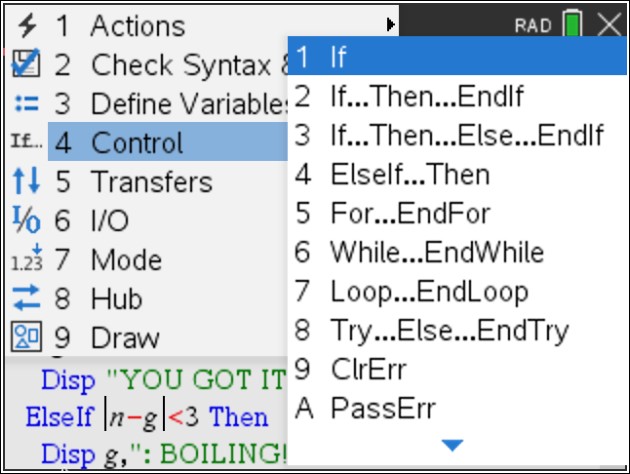
Step 3
To start a new program:
- From the Home screen, select New to start a new document
- From the menu shown select Add Program Editor
- Type the name of the program: meme
- Press [enter]
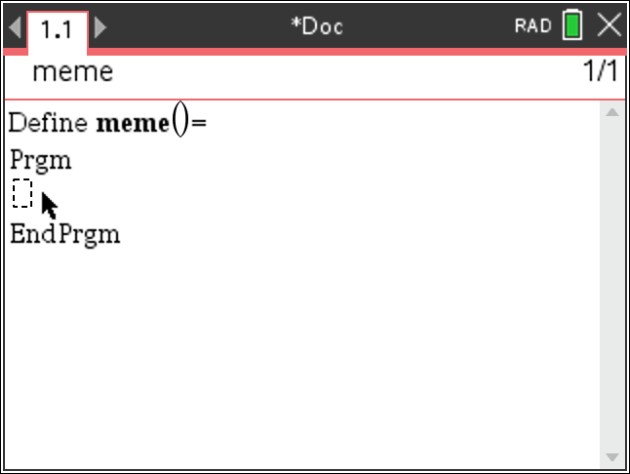
Step 4
When designing a screen for graphics display, the drawing commands are all found on [menu] > [draw].
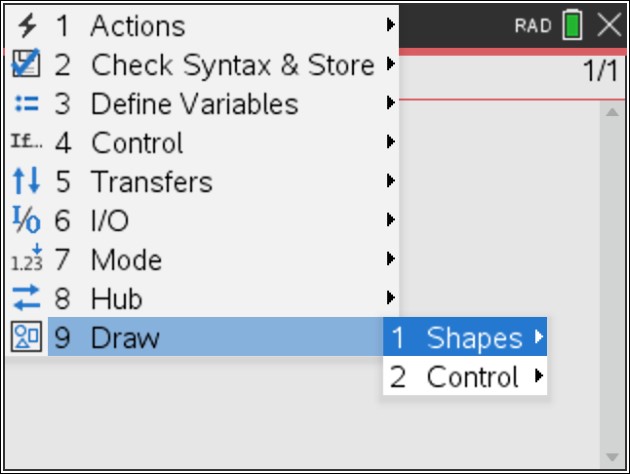
Step 5
When designing a screen for graphics display, the setup commands like SetColor and SetPen are found on [menu] > [draw] > [Control].
The shapes , like FillCircle are on [menu] >Draw > Shapes.
The code to the right (note the numbers entered) draws a large yellow dot when you run the program by pressing ctrl-R and press [enter].
Examples: SetColor red_value, blue_value, green_value
values between 0 and 255
FillCircle x-center, y-center, radius
values depend on window setting
Does your image remind you of anything?
For a refresher on more Draw tools, see Unit 6 of the TI-Nspire™ CX II technology lessons at TI Codes.
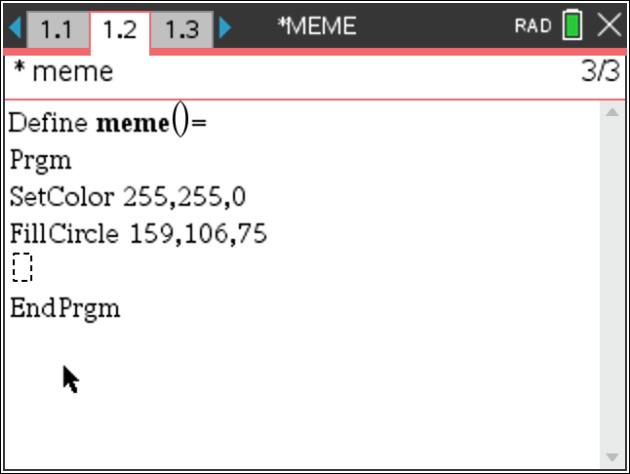
Step 6
To draw text (words) on the graphics screen use
DrawText x, y, ”message”
Found on [menu] > Draw > Shapes.
If you use the two commands (SetColor 0,0,0 is black) in the image to the right, you will see the black word
SMILE!
starting at the pixel in column x=140, row y=197 of the graphics canvas when you run the program.
Recall that the screen is 318 pixels wide and 212 pixels high and that the default window has (0,0) in the upper left corner of the screen. Change the numbers in the DrawText command to see where the message is drawn.
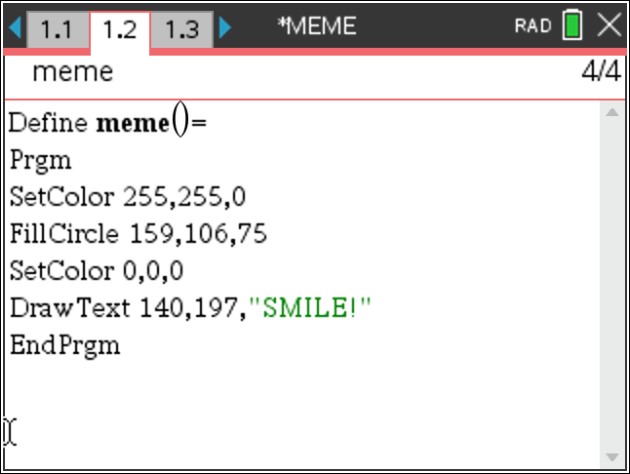
Step 7
Other Draw commands are on [menu] > Draw > Shapes:
- DrawLine a, b, c, d draws a segment between the points (a,b) and (c,d)
- DrawCircle a, b, r draws a circle with center at (a,b) and a radius r
Both of these commands depend on the current window setting for the coordinates. Other commands on the Draw menu have similar arguments
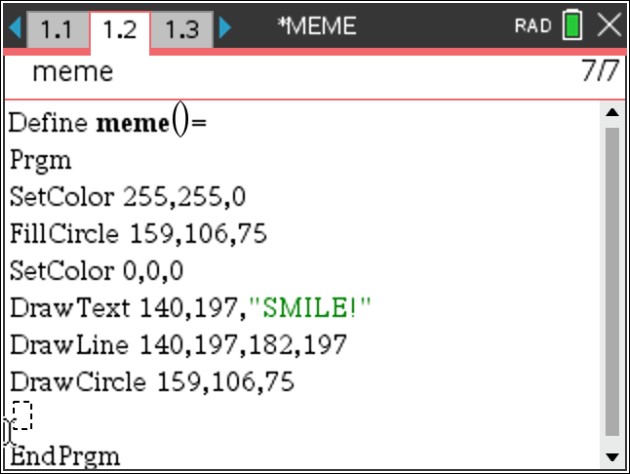
Step 8
If you have entered the code presented so far, and run the program, you will see the screen shown to the right. Note the SetColor controls as well.
When designing your meme screen, you will be adjusting the numbers in these command a lot to get things just right. The numbers used here,
DrawLine 140, 197, 182, 197
were found by “guess and check” to get the word SMILE! underlined just right.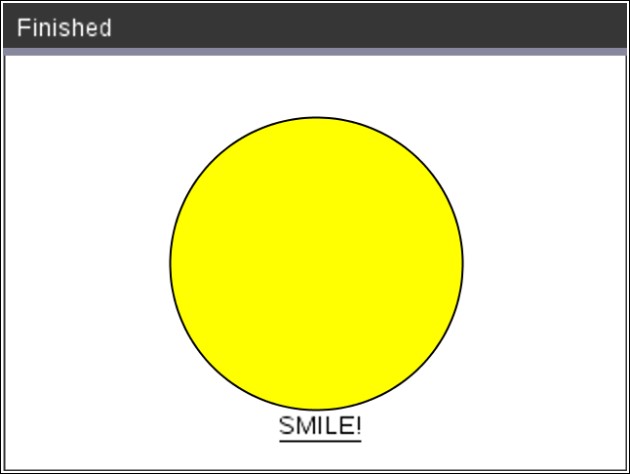
Step 9
Individual points can be plotted using the command
PlotXY x, y, style
PlotXY 0, 0, 1 makes a dot in the upper left corner of the screen. It may be hard to see, so try some other coordinates.
PlotXY 159, 106, 1 makes a dot in the center of the screen.
The third value (1 in these examples) is the style of the point and can be a number from one to 13. Try them all.
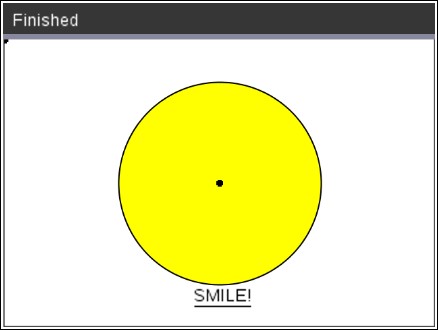
Step 10
DrawArc and FillArc are a little trickier. We first make a rectangle (surrounding the arc) and you will have an idea:
DrawRect 50, 50, 200, 100
DrawArc 50, 50, 200, 100, 180, 180
The arc is inscribed in the rectangle, just touching each side. The last two values (180, 180) are the StartAngle and the ArcAngle to produce the lower portion of the ellipse shown. It starts at 180 degrees (“west”) and goes another 180 degrees counterclockwise. A complete circle or ellipse can have any StartAngle and should have an ArcAngle of 360 degrees.
You can draw the arc without drawing the rectangle. Try other values for StartAngle and ArcAngle.
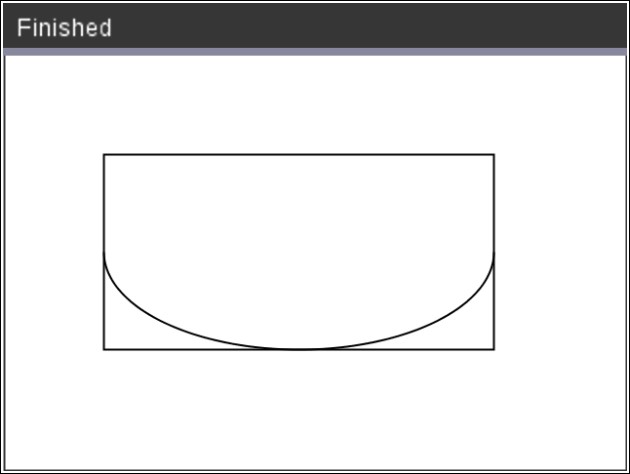
Step 11
You can draw the arc without drawing the rectangle. We used SetPen 2,1 to make a thick arc.
Try your own values for the bounding rectangle, the StartAngle and the ArcAngle. Positioning the arc just right is also a matter of guessing well!
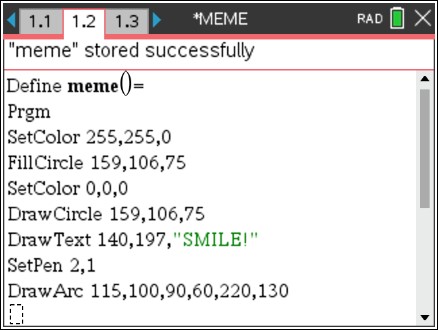
Step 12
How about that “Mona Lisa smile”?
Our smiley face meme needs eyes and a better message. That will be left up to you using two FillArc commands.
Or … create your own meme!
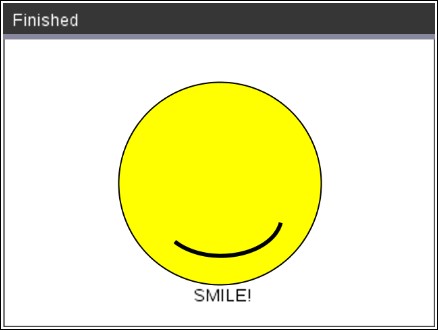
Step 13
Share your project.
You can take a picture of your calculator screen to share with the world!
Share it on social media, and tag it @TICalculators.
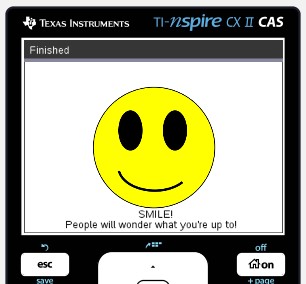
Step 14
Here is a sample TI-Nspire™ CX II graphing calculator TI-Basic program and its result:
Step 15
With this start you can complete the smiley face display or create a meme of your own design.
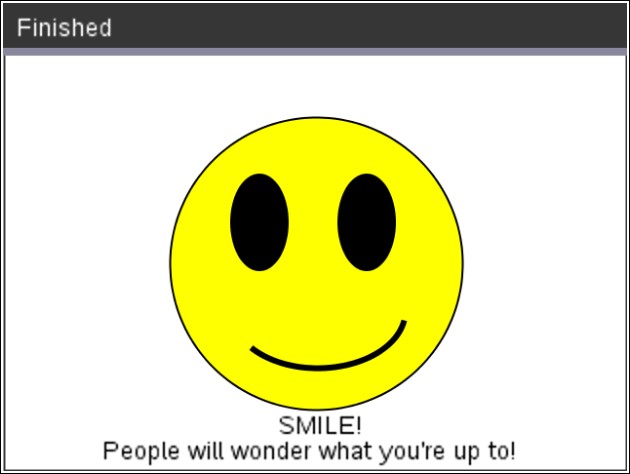
Step 16
Congratulations!
You have completed the requirements for earning your Cadette Coding for Good, Badge 1: Coding Basics. Now that you have completed this requirement, you are challenged to give service by sharing what you have learned about coding with others. Refer to the Coding for Good Girl Scout guide for suggestions on how to do so.
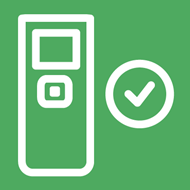
Step 17
What’s next? (Optional extensions)
Ready to try some additional practices with your new skills?
- With the skills you learned from TI Codes, and these additional ideas, you can probably figure out how to write a program to draw the smiley face, the original emoji (or an emoji of your own design).
- After completing Unit 6 of the TI Codes lessons, you might be ready to create an animation on your TI-Nspire™ CX II graphing calculator. Use the Clear command on the Draw > Control menu to erase the screen, and use a loop to draw objects in different locations on the screen.
- See the Basketball Game in the Beyond Basics section of TI Codes. These projects are designed using TI-Basic on a TI-84 Plus family graphing calculator, but since the languages are so similar you should be able to “translate” them to TI-Basic on the TI-Nspire™ CX II graphing calculator.
Be sure to share your work on social media and tag it @TICalculators!
Senior: Coding for Good, Badge 1 >>
Introduction
The programming language BASIC (stands for Beginner’s All-purpose Symbolic Instruction Code) was developed in the 1960s as an easy system for teaching computer programming. TI-Basic is similar to other flavors of BASIC, but you must select the programming words and commands from the onboard menus, as you will soon see.
The Senior Level, Badge 1: Coding Basics consists of two separate programming projects:
- A graphics program to produce a self-portrait, and
- A quiz program to help someone learn or review facts or a skill
The TI-Nspire™ CX II graphing calculator is required at this level.
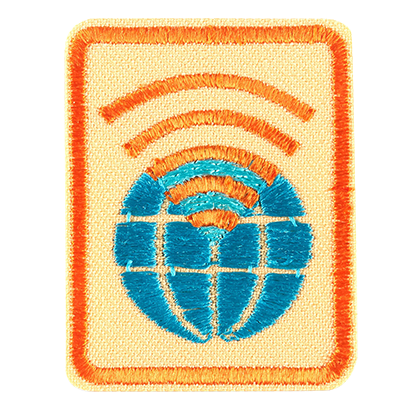
Requirements
Senior Coding for Good, Badge 1 requirements:
Self-portrait
1. Use functions to create a self-portrait.
2. Write code to create a portrait.
Quiz
1. Learn about computer logic.
2. Explore “If” statements.
3. Use computer logic to create a quiz show.
Be sure to review the “Senior Coding for Good” overview/guide for the badge requirements and badge steps provided by your leader and the Girl Scouts. It also includes relevant vocabulary and interesting background information to spark your interest in coding. This lesson will allow you to earn Badge 1: Coding Basics, using your TI-Nspire™ CX II graphing calculator
Step 1
For an introduction to programming on a calculator TI-Nspire™ CX family graphing calculator, see the TI Codes lessons. Units 1 through 4, and Unit 6 (Drawing), should be enough to get started, including coverage of algorithms and conditionals as the Badge requirements state. After completing those lessons, you will then have a basic understanding and can return to this lesson for the Senior Coding, Badge 1 project. Note: You will follow the step-by-step instructions given on the webpages, but will actually do the coding on your calculator.
How to navigate: You will navigate through each Skill Builder by clicking on the “Step 1” in the right bottom corner. After you complete all the steps in Skill Builder 1, you will then move to Skill Builder 2, and so on until you complete all of Unit 1. You will then move on to Unit 2, 3, 4 ... and do the same thing.
Step 2
After you have completed the TI Codes units you know about:
- The programming process (planning, coding, testing and debugging)
- Using the TI-Nspire™ Basic Program Editor and its menus
- Running a program
- Some important pieces of TI-Basic code including: Request, Disp, variables and assignment statements, If…Then…Else structures and loops, like For and While (some of these statements found on the [menu] key are shown to the right)
- Draw features in the programming world of TI-Nspire™ CX II
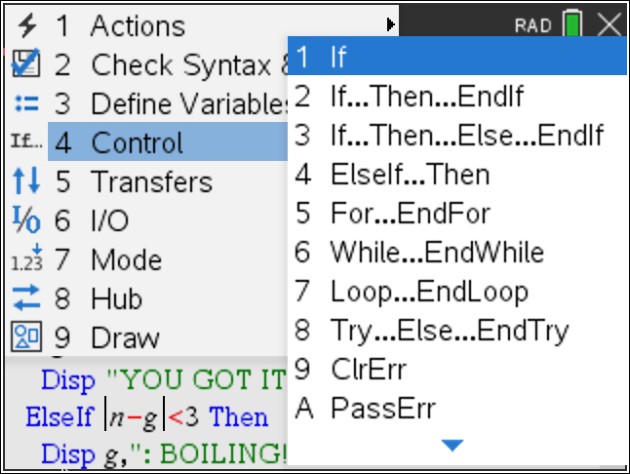
Step 3
Part 1: Self-portrait. Plan your project on paper first!
There are several functions on the [menu] > Draw > Shapes menu shown to the right that let you design interesting graphic images.
These tools are covered in Unit 6: Drawing of the TI Codes lessons you studied.
These functions produce points, lines, rectangles, circles and ellipses, polygons and text in the colors of your choosing
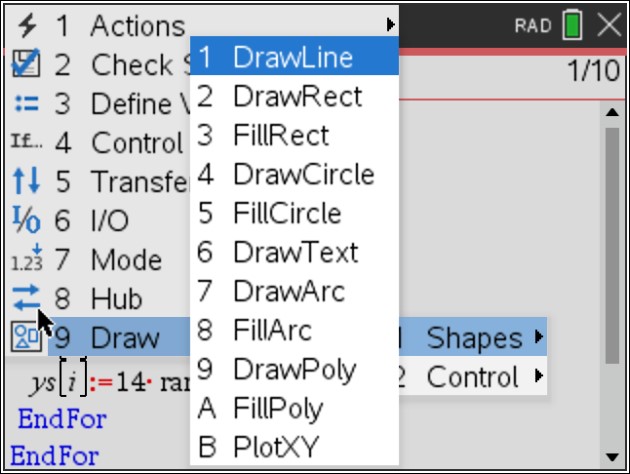
Step 4
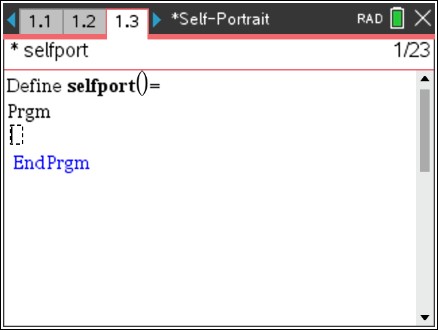
Step 5
To refresh these skills, use the statement
DrawLine x1, y1, x2, y2
to draw the segment between the points (x1, y1) and (x2, y2)
Example: DrawLine 50, 30, 250, 200
Run the program by pressing [ctrl] -R and then [enter] and you see a diagonal segment on the screen.
Note: To also display the two pairs of coordinates as shown here, that is another command. They are shown here for location purposes only.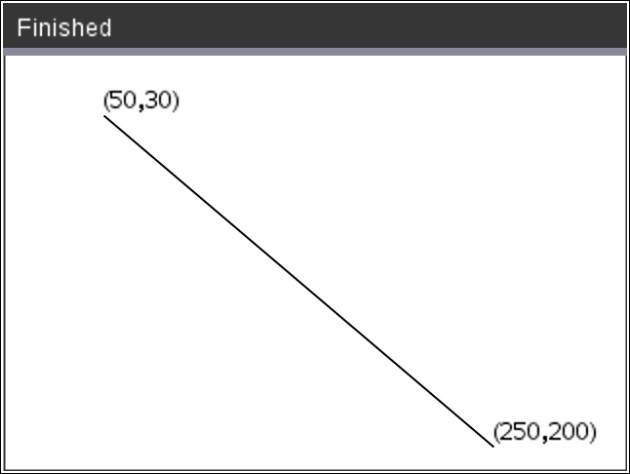
Step 6
To return to the Program Editor, press any key to remove the graphics canvas and return to the Calculator app (shown).
Either press [ctrl]-[leftarrow] or use the mouse to click on the previous page tab at the top of the screen (page 1.3 in the image).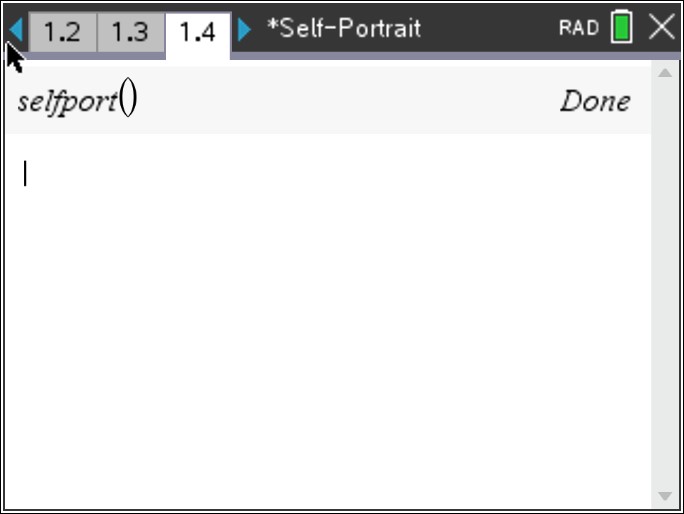
Step 7
To draw a red rectangle:
SetColor r, g, b
( r, g and b must be integers between 0 and 255)
DrawRect x, y, width, height
(x, y) is the location of the upper left corner
Example: SetColor 255, 0, 0
DrawRect 80, 60, 70, 70
Run the program by pressing [ctrl] -R and then [enter]. It Makes the red square in this image since the width and height are both equal to 70.
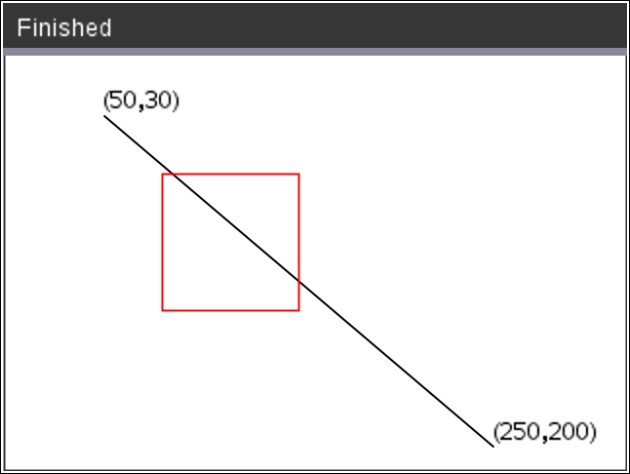
Step 8
Let’s try this. To make a solid (filled) circle,
FillCircle x-center,y-center, radius
Example to make a big yellow dot:
SetColor 255, 255, 0
FillCircle 250, 70, 50
Note: The center is (250, 70) and the radius is 50.
Now run the program again. Does yours look like this?
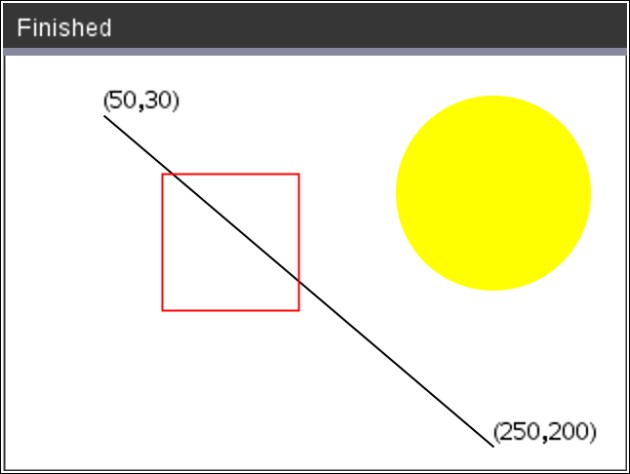
Step 9
Now let’s try and add some text on the screen:
DrawText 30, 50, ”Hello”
Note that (50,30) (the line’s endpoint) and (30,50) (“Hello” position) are two different locations on the screen.
Draw the word Hello at the coordinates (30, 50). The coordinates are the lower-left corner of the text area. Is your “Hello” yellow? Use SetColor to change the color before the DrawText command.
This is how the coordinates were displayed earlier:
DrawText 50, 30, "(50,30)"
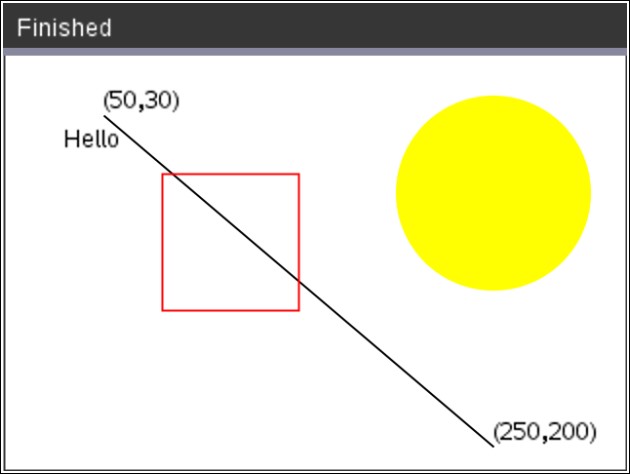
Step 10
DrawArc and FillArc are a little trickier. (We started a new program for this demonstration but you can continue with your program and add to your graphics.)
First make a rectangle (surrounding the arc), and you will get the idea:DrawRect 50, 50, 200, 100
DrawArc 50, 50, 200, 100, 180, 180
Drawing an arc is like drawing a rectangle. The arc is inscribed in the rectangle, just touching each side. The last two values (180, 180) are the StartAngle and the ArcAngle to produce the lower portion of the ellipse shown. It starts at 180 degrees (“west”) and goes another 180 degrees counterclockwise.
A complete circle or ellipse can have any StartAngle but should have an ArcAngle of 360 degrees. So, the DrawArc/FillArc commands need a “bounding rectangle” and an angle interval to make an arc. If the rectangle is a square then the arc will be part of a circle, otherwise it is part of an ellipse.
You can, of course, draw the arc without drawing the rectangle.
Try experimenting with other values for StartAngle and ArcAngle.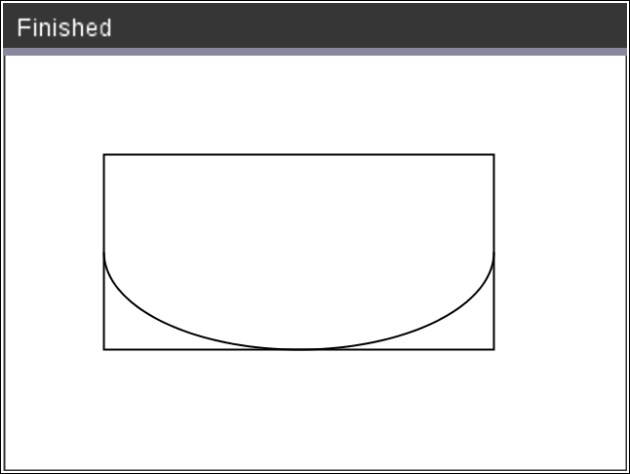
Step 11
To draw a filled or outlined polygon (or sequence of segments, a “poly-line”) create two lists, one for x-values and one for y-values:
xs:={10,20,30,20,40}
ys:={10,10,20,30,40}
then use the DrawPoly command:
DrawPoly xs,ys
Reminder: := is found on [ctrl]-[math templates] to the right of the 9 key.
Notice that there are five points which makes the four segments in the upper left corner of the screen.
Note: The word “Hello” appears in a different location in this image. Can you imagine how that happened?
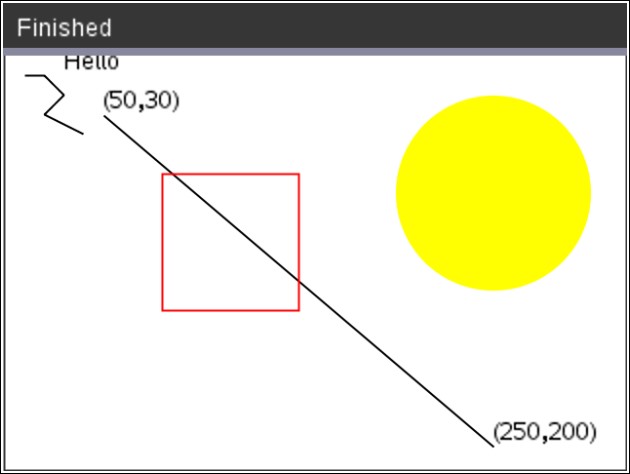
Step 12
A filled polygon is useful for drawing large portions of your self-portrait.
Example to draw some brown hair:
SetColor 210,105,30
Note: The following two lists will not fit on your screen. As you type the values, your screen will shift. Just keep typing and press [enter] at the end.
xs:={187, 206, 208, 191, 183, 181, 173, 138, 128, 131, 113, 93, 98, 121, 161, 166, 169, 188}
ys:={67, 99, 134, 141, 137, 105, 88, 88, 105, 139, 146, 131, 93, 67, 60, 71, 60, 67}
FillPoly xs,ys
Run your program. Does it look like this? You are learning very useful tools. You will soon be ready to draw your own “self-portrait.”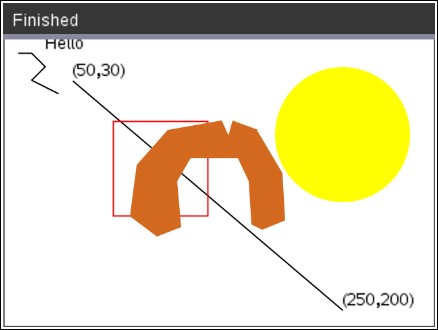
Step 13
Now, it’s your turn! Write your own graphics program using a combination of Draw commands to create your own self-portrait program.
All you have to do is determine the correct x- and y-coordinates. Remember: Plan first, code later. Graph/grid paper will help.
Hint: the screen coordinates are: upper-left (0,0) → lower right: (317,211). On ordinary graph paper (1/4" grid lines) you might want to use a scale of 10 pixels per grid unit.
Share your project on Instagram, or other social media, and be sure to tag it @TICalculators.
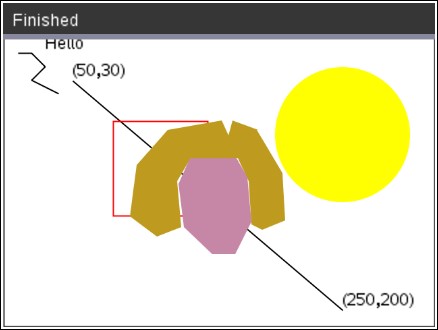
Step 14
Part 2: Quiz
A “Quiz program” presents the user with a series of questions to answer. In this sample project we use a true/false format. The program keeps track of the number of correct answers and reports a score at the end of the program. To prepare for our program we will store text “strings” in lists on the TI-Nspire™ CX II graphing calculator. Set up two lists, one containing questions and the other the answers.
In the screen to the right, in the Lists & Spreadsheet app, we made a list called qs (questions) and a list called as (answers: true or false). Each question and each answer is entered into the spreadsheet cells using “quotation marks.” See the status line at the bottom of the image.
The list names (qs and as) are in the TOP cell above the lists and the formula cell (the second row on the screen) is left empty.
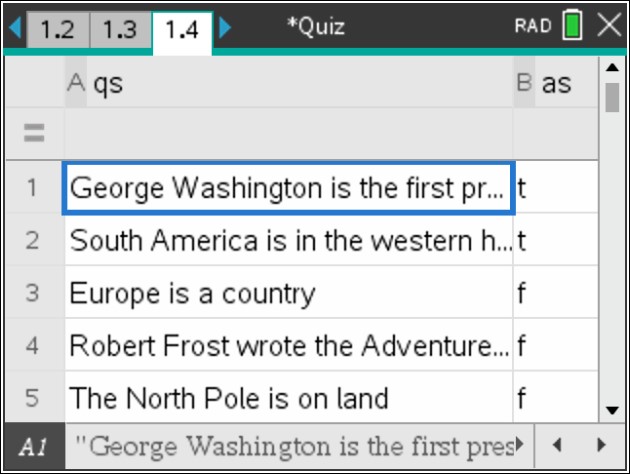
Step 15
The plan
Use a For loop to process each question qs[k]. Use DispAt statements to position each part of the question on the screen. Keep track of the number of correct answers. Report the score at the end of the quiz.
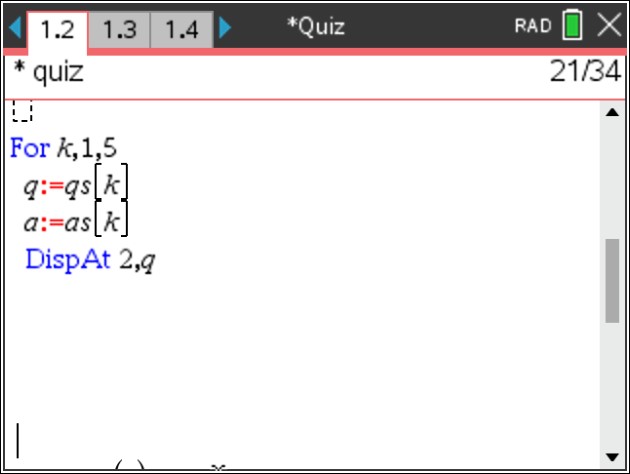
Step 16
Start a new document ([home] New), and Add Program Editor from the new page menu. Name the program quiz.
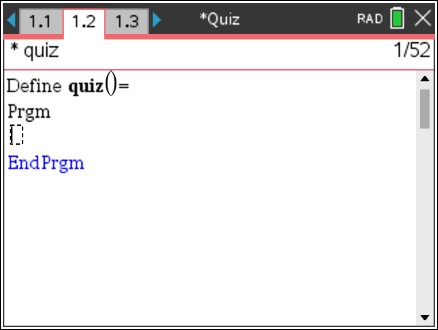
Step 17
The variable counter is set to 0 to count the correct answers.
A preliminary For loop is used to clear the screen:
For k, 1, 8
DispAt k, “ “
EndFor
Hints: [menu] > Control> For…EndFor
[menu] > I/O > DispAt
Indentation is provided and recommended but optional.
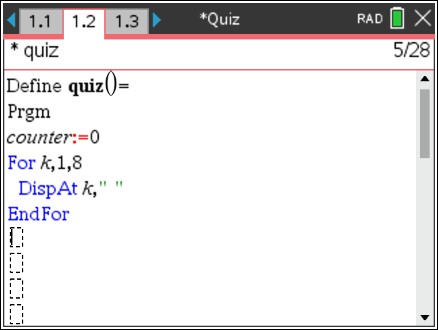
Step 18
Now begin the main part of the program. After the clear screen loop from the previous step write another For…EndFor loop ([menu] Control) that will:
- Display the question (and answer choices if multiple-choice). The statement q := qs[k] stores the kthquestion in the variable q. Then the DispAt statement ([menu] I/O) displays the question on line 2.
- Pause the program using getKey(1) ([menu] I/O) (user presses any key to continue).
- Input the answer using RequestStr ([menu] I/O) (user presses enter to answer).
- See if the answer is correct and count the correct answers. (This code is missing in the image to the right.)
Note: The first few steps are shown here, but this is not the complete program so, although the program runs, there is more to do:
- Add an If statement in the loop to see if the answer is correct and count the number of correct answers
- Add statements below the loop to report the final score
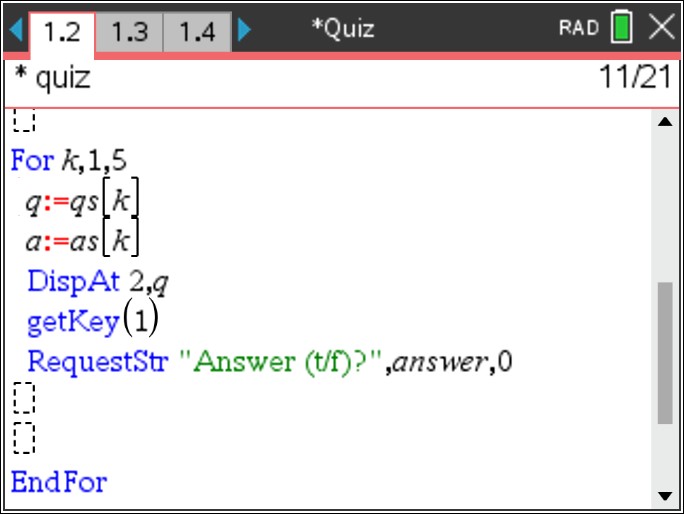
Step 19
The statement getKey(1) pauses the program until a key is pressed (see screen to the right) to give the scout ample time to study the question before answering. getKey() is on [menu] I/O.
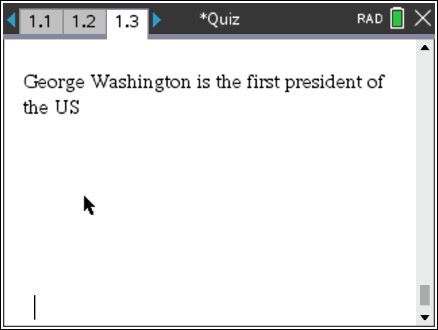
Step 20
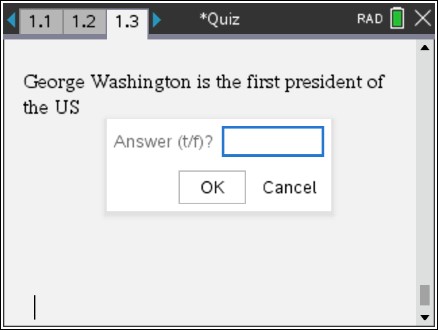
Step 21
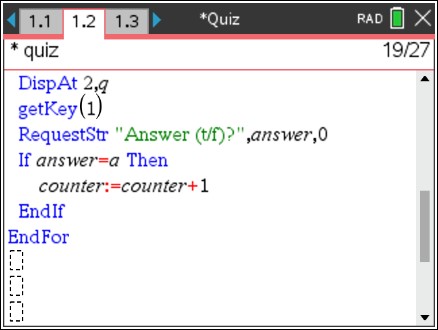
Step 22
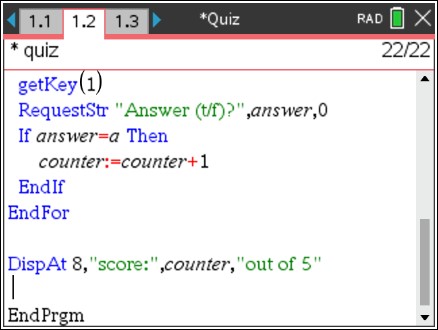
Step 23
When you run the program (press ctrl-R) each question is displayed one at a time. Press a key to see the input box, type an answer and [enter].
At the end of the quiz the score is displayed.
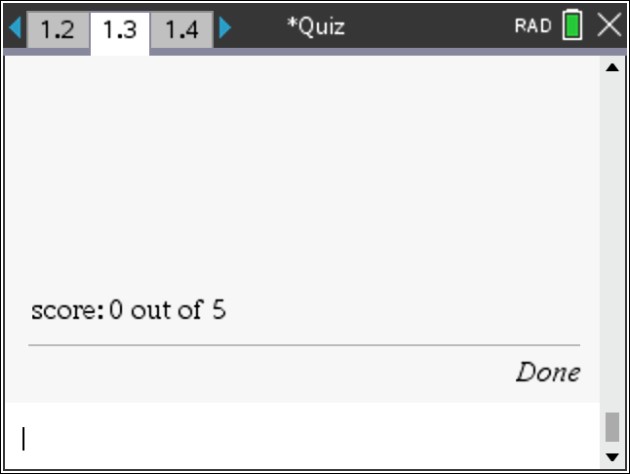
Step 24
Using this program as a sample, you can improve the project in many ways. Get creative! How about a quiz about the Girl Scouts? 😊
After your program is finished, and before sharing the file with others, you can remove the Lists & Spreadsheet app to hide the questions and answers from the user. On the Lists & Spreadsheet app, press ctrl-K to select the app, then press [del] to remove the app from the document. The lists will remain in the document.
In the future, you can always insert a new Lists & Spreadsheet app again, and add the stored lists to the spreadsheet to edit the questions and answers.
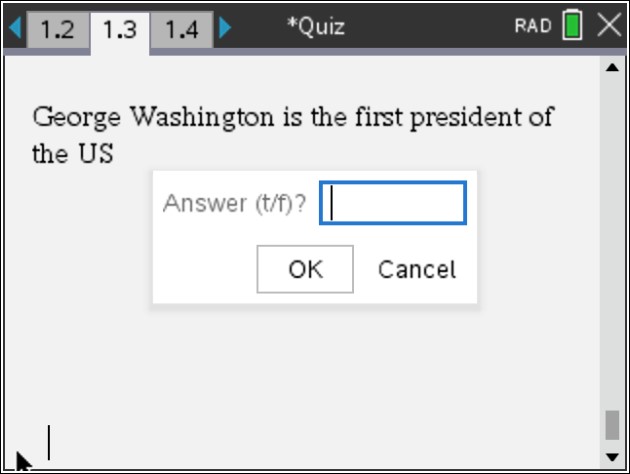
Step 25
Congratulations!
You have completed the requirements for earning your Senior Coding for Good, Badge 1: Coding Basics. Now that you have completed this requirement, you are challenged to give service by sharing what you have learned about coding with others. Refer to the “Coding for Good” Girl Scout guide for suggestions on how to do so.
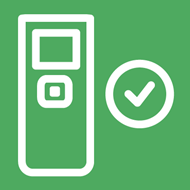
Step 26
What’s next? (Optional extensions)
Ready to try some additional practices with your new skills?
1. In the Quiz project:
- Create a multiple-choice quiz with three to five choices for each question. The correct answers will be the letters a, b, c ….
- To set up a multiple-choice quiz using three-to-five-answer choice lists such as, bs, cs, ds, es, then, in your program ask the user to enter the letter of their choice.
- Offer multiple tries (“Wrong, try again.”); give full credit for getting it right on the first try and give partial credit on later tries. This will take an extra loop in the response section of the program.
- Display a percent score at the end.
- Display the questions in a random order.
Ambassador: Coding for Good, Badge 1 >>
Introduction
The programming language BASIC (stands for Beginner’s All-purpose Symbolic Instruction Code) was developed in the 1960s as an easy system for teaching computer programming. TI-Basic is similar to other flavors of BASIC, but you must select the programming words and commands from the onboard menus, as you will soon see.
The TI-Nspire™ CX or TI-Nspire™ CX II graphing calculators can be used at this level.
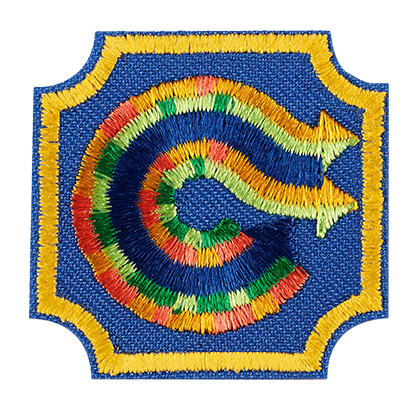
Requirements
Ambassador Coding for Good, Badge 1 performance:
Song lyrics; sound using the TI-Innovator™ Hub
1. Learn about functions through song lyrics.
2. Learn about loops through song patterns..
3. Write an algorithm duet.
4. Code a performance routine.
5. Share your coded routine with others.
Be sure to review the “Ambassador Coding for Good” guide for the badge requirements and badge steps provided by your leader and the Girl Scouts. It also includes relevant vocabulary and interesting background information to spark your interest in coding. This lesson will allow you to earn Badge 1: Coding Basics using your TI-Nspire™ CX or TI-Nspire™ CX II graphing calculator.
Step 1
For an introduction to programming on a calculator TI-Nspire™ CX family graphing calculator, see the TI Codes lessons. Units 1 through 4 should be enough to get started, including coverage of algorithms and conditionals as the Badge requirements state. After completing those lessons, you will then have a basic understanding and can return to this lesson for the Ambassador Coding, Badge 1 project. Note: You will follow the step-by-step instructions given on the webpages, but will actually do the coding on your calculator.
How to navigate: You will navigate through each Skill Builder by clicking on the “Step 1” in the right bottom corner. After you complete all the steps in Skill Builder 1, you will then move to Skill Builder 2, and so on until you complete all of Unit 1. You will then move on to Unit 2, 3, 4 ... and do the same thing.
Step 2
After you have completed the TI Codes units, you know about:
- The programming process (planning, coding, testing and debugging)
- Using the TI-Nspire™ Program Editor and its menus
- Running a program
- Using the TI-Innovator™ Hub (optional)
- Some important pieces of TI-Basic code: Input, Disp, variables and assignment statements, If…Then…Else structures and loops, like For and While (some of these statements are shown to the right)
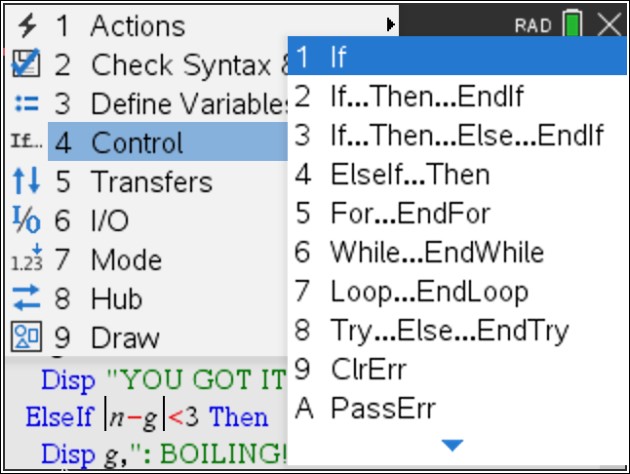
Step 3
The sample program for this lesson uses the lyrics of the song “She Loves You” by John Lennon and Paul McCartney (of the Beatles). Do not begin coding yet.
The basic structure of songs is the verse, chorus and refrain structure. When a portion of a song is repeated (like the chorus or refrain), then it is a perfect opportunity to incorporate a subroutine in computer programming. The main program in the image to the right contains and “calls” the subroutine chorus() to repeatedly display the refrain or chorus at the proper times without having to rewrite the lyrics.
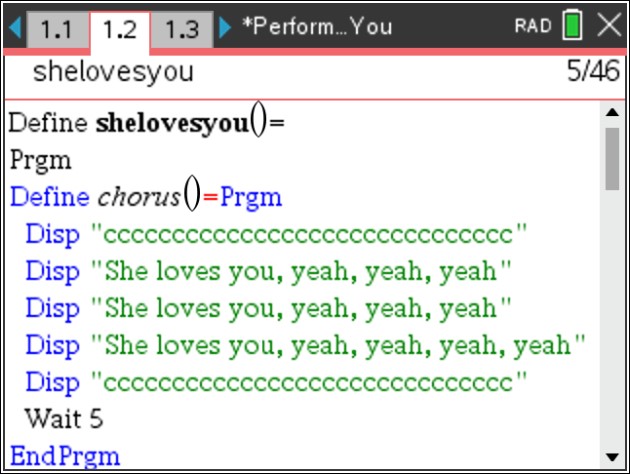
Step 4
This lesson explains how to write and use subroutines on a TI-Nspire™ CX family graphing calculator.
Notice that in this image, the main program shelovesyou() contains the subroutine chorus() along with another subroutine called refrain().
To write a program with subroutines, follow the next steps.
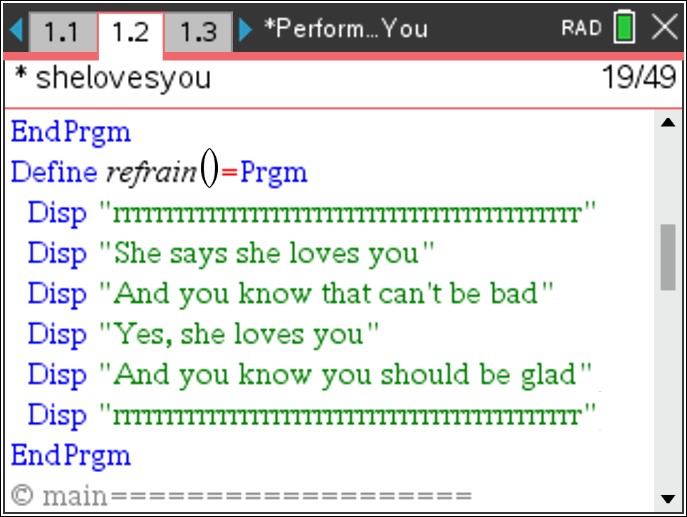
Step 5
Start the main program by making a new document ([home] New). Select Add Program Editor from the next apps menu. We named our program shelovesyou.
The subroutines are created first:
- Press [menu] > Define variables, and select the word Define from the menu.
- Right after the word Define, write the name of the subprogram, chorus( )= including the parentheses and the equals sign. Do not press [enter] yet.
- Press [menu]> Define Variables and select the structure Prgm…EndPrgm.
Tip: When defining a subroutine within a program it is important that the keyword Prgm be on the same line as Define chorus()=Prgm. An error occurs if it appears on the next line as it does for the main program.
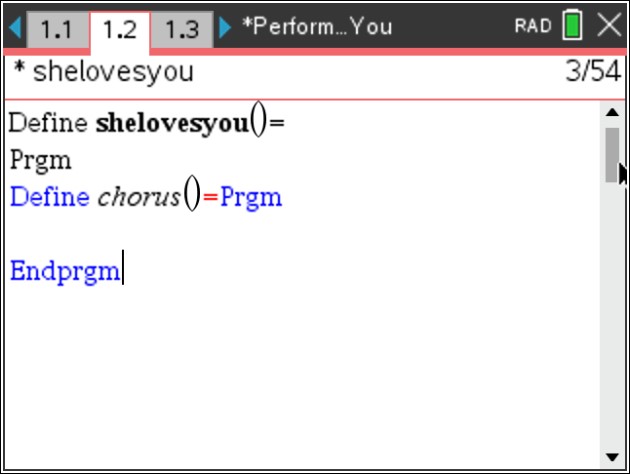
Step 6
In the chorus subroutine write the Disp statements (found on [menu] I/O) that show the lines of the chorus portion of the song now. Or you can save that for later.
The two lines that display “ccccccccccccccccccccccccc” are for programmer information only, indicating that this section of text is the chorus. These can be removed (or commented) later, but for coding purposes it makes the program execution clear.
Tip: To speed up the typing, you can select, copy and paste code just like on a computer: Use shift-arrows to select, ctrl-C to copy and ctrl-V to paste.
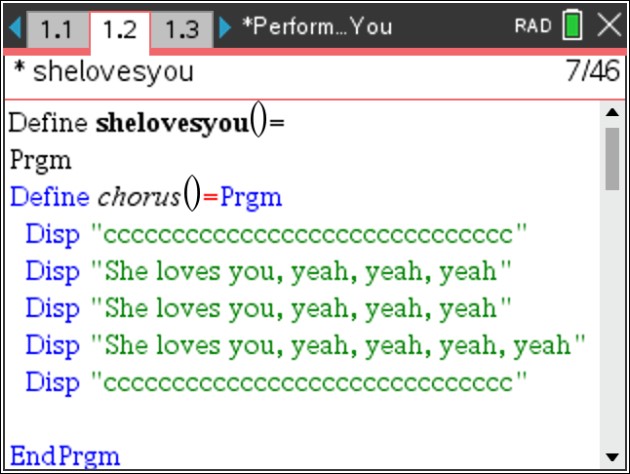
Step 7
This sample song also contains a refrain. Below the chorus subroutine (right after the EndPrgm of the chorus section), start another subprogram called refrain().
In this subroutine, Display the lyrics of the refrain (not shown).
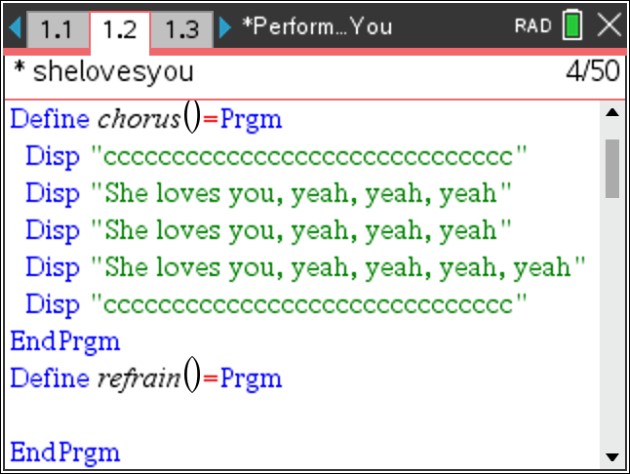
Step 8
After the refrain() subroutine (and any other subroutines your song may use), you can begin writing the main program consisting of the verses.
This sample song begins with the chorus(), then verse 1 (note the © comment), then the refrain().
Comments (the lines beginning with the © symbol) are used a lot in programming to keep the code organized and clear to the reader of the code. These comments are not displayed when the program is run.
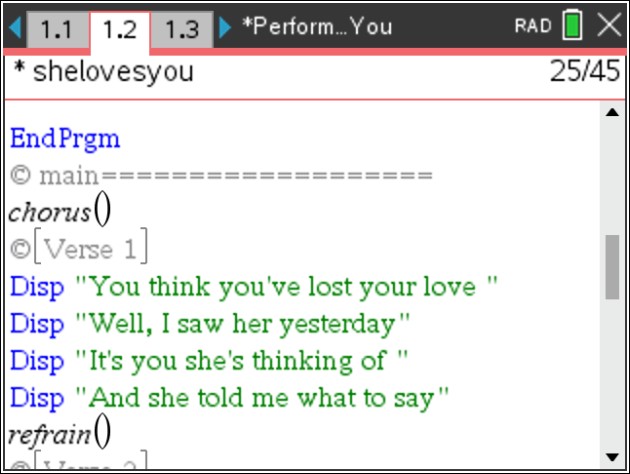
Step 9
You may want to add some Wait statements to your program in appropriate places to control the display so that the lyrics don’t scroll by too fast.
Wait is found on [menu] > Control (at the bottom) and on [menu] > Hub. It needs a number of seconds to wait after the command. Wait 5 means “wait 5 seconds.”
Share your project on Instagram, or other social media, and be sure to tag it @TICalculators.
Step 10
Congratulations!
You have completed the requirements for earning your Ambassador Coding for Good, Badge 1: Coding Basics. Now that you have completed this requirement, you are challenged to give service by sharing what you have learned about coding with others. Refer to the “Coding for Good” Girl Scout guide for suggestions on how to do so.
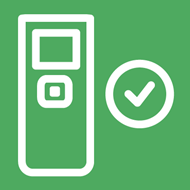
Step 11
What’s next? (Optional extensions)
Ready to try some additional practices with your new skills?
1. Want to try and include some audio? We can use the speaker on the TI-Innovator™ Hub, write a song that includes using the Send “SET SOUND…” command with the proper notes (or frequencies) and timings (Wait).
Perhaps you can even sync the sounds with the lyrics to make a karaoke machine!
The Send and Wait statements (shown) are incomplete, and the code for producing notes is more involved than this.
If you plan to use the TI-Innovator™ Hub for sound, then also check out the TI-Innovator™ lessons at 10 Minutes of Code.
If you are using a TI-Nspire™ CX II graphing calculator, consider using the graphics canvas and DrawText statements to display your lyrics instead of the Disp statements used in this lesson. See Unit 6 of the TI Codes materials for more information on the Draw commands.
Step 12
Optional extensions (continued)
2. The Beyond Basics section of TI Codes for the TI-84 Plus family graphing calculator provides several interesting programming projects. In keeping with the theme of this badge, try the Piano project or the Musical Scale project. Both make use of the TI-Innovator™ Hub for making sounds. You should be able to adapt the projects from the TI-84 Plus family graphing calculator to the TI-Nspire™ CX II graphing calculator, since the TI-Basic languages are the same but the implementation (keywords, punctuation and syntax) are slightly different.
Make your programs more efficient using subroutines/subprograms as described in this lesson to play the different sections of your songs.
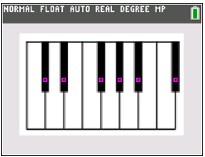

The TI expert in your area is available to make sure Girl Scouts have everything they need to earn their first Coding for Good badge.

If you have questions about getting started, the Girl Scouts Volunteer Guide helps explain what you can do to get your Girl Scouts coding.

Learn even more about how an TI expert can help Girl Scouts create content-rich, valuable learning experiences with coding and STEM activities.